Post Graduate Program in Full Stack Web Development
Enroll in Tech-Lync’s Full Stack Web Development Course and transform from a novice to a proficient developer, even without prior coding experience. This comprehensive course guides you through the fundamentals to advanced concepts, culminating in the creation of a robust eCommerce platform from scratch.
Program Overview
- The course offers comprehensive knowledge on building a website from the ground up.
- This course also emphasizes establishing a solid foundation in Java programming, as well as data structures and algorithms.
- In this course, you will be introduced to essential software tools such as HTML5, CSS, Bootstrap 4, and Spring MVC. Each topic will be covered with practical examples, providing you with hands-on experience to reinforce your learning and ensure you can apply these technologies effectively in real-world scenarios.
- The course includes hands-on projects, such as developing an e-commerce website, to prepare students for industry standards.
Syllabus
On a daily basis we talk to companies to fine tune our curriculum. Here are the list of courses that are part of this program
Why enrol in the Program?
- A complete understanding of frontend and backend development involves mastering the design and user experience aspects of a website or application (frontend) alongside the server-side logic, database interactions, and application architecture (backend), and seamlessly linking them through APIs, middleware, and efficient data handling to create a cohesive, functional, and responsive digital product.
Practical experience with the software and technologies used in full stack development.
The student can get employed as:
- Full-Stack Developer
- Back-End Developer
- Front-End Developer
- Web Developer
- Web Designer
Course Syllabus
Frontend Track
On a daily basis we talk to companies expert in these track to fine tune our curriculum. In total, there are 6 courses that are available in this track
Course 1 - The Complete Front-End Development - 2.0
Module 01 – HTML5
- The topics covered in this module are:
- Valid document structure, anatomy of HTML syntax
- HTML5 semantic tags – Elements and attributes, Block and inline elements
- Essential HTML5 tags – headings, paragraph, styles, comments, colours
- HTML lists – unordered and ordered lists
- Inserting images using HTML
- How to create hyperlinks using anchor tags
- HTML tables
- HTML forms
- HTML Div – Layout and separate content for CSS styling
- Classes and ID
- HTML iFrames
- HTML Graphics
- HTML Media
- HTML APIs- Geolocation, Web Storage, Web Workers, Drag and Drop, SS Event
- HTML best practices- HTML vs XHTML”
Module 02 – CSS
- In this module, you will learn:
- Basics of CSS3, CSS rules
- Comments, Colors, background, border, margin, padding, height, and width
- CSS selectors and properties
- Inline, internal, and external CSS
- Font styling, web safe fonts – Texts, icons, links
- The ‘Box model’
- CSS sizing methods
- CSS static, relative, and absolute positioning systems
- CSS float and clear
- Pseudo classes and pseudo elements
- Class vs. ID
- Opacity, Navigation bars, Dropdowns
- CSS rule conflict resolution. specificity, and implementing style hierarchy
- Images and forms styling
- Transitions, animations, pagination, 2D and 3D transforms
- Responsive web design
- The grid system
- Flexbox, media queries
- CSS coding best practices
Module 03 – Bootstrap
- In this module you will learn:
- The mobile-first paradigm
- Wireframes in the design phase
- Twitter Bootstrap – Grid System
- How to install the Bootstrap framework
- Bootstrap containers to layout website easily
- Other Bootstrap components
- Bootstrap carousels
- Bootstrap cards
- Bootstrap navigation bars
- Bootstrap themes
- Font-awesome
Module 04 – Page Events
- The topics covered in this module are:
- ECMAScript
- JavaScript vs Java
- Popularity and Importance
- Basics of JavaScript
- JS Statements
- JS functions
- Adding JS to your website
- Document Object Model
- Manipulating DOM
- Events
- Exception Handling
- Forms and JS
Module 05 – JavaScript
- The topics covered in this module are:
- Variables, data types, and operators in JavaScript
- Statements, syntax, comments, and events
- Loops, control, and conditionals
- JS forms
- Document Object Model (DOM)
- Browser Object Model (BOM)
- JS functions
- Scope and closures
- Object-Oriented Programming (OOP)
- JS objects and prototypes – keyword
- Cookies
- Exception handling
- Arrow functions
- Promises
- Template string, default parameters, spread and rest operator, destructuring, generators, Set, Map, WeakSet, WeakMap
- HTML APIs
- Separation of concerns, refactoring, debugging, and coding best practices
Module 06 – AJAX, JSON and XML
- We will be covering
- Serving and retrieving data to a website
- Asynchronous loading – Set up and handle AJAX requests and responses
- JSON
- XML
- Processing JSON data
- JSON vs. XML
Course 2 - Angular Web Development for the Real World
Week 01 – Course Overview, Prerequisites, and Introduction to Frontend Web Development
- Introduction to the course
- Course prerequisites and how to learn them better
- Types of application and developers
- Difference between frameworks and libraries
- What is in Angular?
- Introduction to TypeScript
Week 02 – Introduction to Angular Framework and Architecture
- Why Angular?
- Features in Angular
- History of Angular (AngularJS vs Angular)
- Angular Architecture
- Building blocks of Angular
- How to think in Angular
- Getting started with a basic Angular app
- Project structure best practices
- Overview of our course project
Week 03 – Understanding Modules and Components
- Understanding modules vs components vs directives
- Lazy loaded modules
- Data Binding with components
- Property/Event Binding
- Component Lifecycle
Week 04 – Understanding Templates and Advanced Components Concepts
- Templates and View Encapsulation
- Accessing DOM element programmatically
- Dynamic components
- Content projection
Week 05 – Introduction to Services/Dependency Injection Concepts along with RXJS (Reactive Programming)
- Services and dependency injection
- Single vs multiple instances of service
- Reactive programming concept
- Observable vs promise
- RxJS library introduction
- Use cases for Angular services
Week 06 – Intercomponent Communication and External HTTP API Communication using Angular
- Parent-child component communication
- Component communication using services
- Connecting to REST APIs through HTTP/HTTPS
- Handling API response and manipulating it
- Handling errors
- HTTP Interceptors
Week 07 – Understanding Directives and Styling Angular Application with Angular Material
- Structural vs attribute directives
- Built-in directives examples like “ngIf”,”ngFor”,”ngSwitch”, etc.
- Custom directives
- Angular Material introduction
- Features of Angular Material
- Styling Angular app using Angular Material
Week 08 – Understanding Pipes and Introduction to Forms
- Pipes
- Pure vs Impure pipes
- Basic built-in Pipes
- Custom Pipes
- Introduction to forms
- Template-driven and Reactive forms
- When to use which form?
Week 09 – Template Forms
- Creating a basic form
- Accessing form state
- Form validation
- Error handling
- Set values programmatically
- Grouping controls in a form
- Submit/Reset Forms
Week 10 – Reactive Forms
- Creating a basic form
- Accessing form state
- Form validation
- Error handling
- Set values programmatically
- Grouping controls in a form
- Submit/Reset Forms
Week 11 – Introduction to Angular Routing
- Routers – Why and How?
- Navigating with Routers
- Pass and fetch query parameters in routes
- Nested Routes
- Securing routes (Route Guards)
- Use cases for Route Guards
- Routing Strategies
Week 12 – Unit Testing Angular Application and Debugging Code
- How do we test code in Angular?
- How to write unit test cases in Jasmine?
- Writing unit test cases for a few common scenarios in Angular
- How to debug Angular code?
- Tools/utilities to speed up debugging Angular code
Week 13 – Useful Tips and Tricks for Building Real-World Angular Application
- Designing scalable and secure Angular app
- Deployment
- Web Accessibility
- Internationalization
- Angular Universal
- Angular Elements
- Service workers or progressive web app
- Angular Schematics
Course 3 - Data Structures and Algorithms using JAVA
Week – 01 Introduction
A data structure is a named location that can be used to store and organise data. An algorithm is a collection of steps to solve a particular problem. Data structures provide a means to manage large amounts of data efficiently.
- In the first week of this course, you will learn:
- Stack memory vs heap memory in Java
- Physical vs logical data structure
- Abstract data types and data structures
- Complexity analysis
- Asymptotic analysis, comparison of functions, recurrence relations
- Time complexity
- Space complexity
- Master theorem
- Amortized analysis
- Iteration Vs recursion
- Recursion – Factorial, Fibonacci, finding nCr, tower of Hanoi problem.
Week – 02 Arrays, Strings, and Lists
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. A string is a sequence of characters like – “hello“.
- In this session, we will learn about:
- Arrays
- Static arrays
- Dynamic arrays
- 2D arrays, row-major and column-major mapping, matrices
- Strings
- LinkedList
- Singly LinkedList and its operations
- Doubly LinkedList and its operations
- Circular LinkedList and its operation
- Arrays
Week – 03 Stacks and Queues
The stack is a linear data structure that is used to store a collection of objects. It is based on Last-In-First-Out (LIFO).
- In this session, we will be discussing about:
- Stacks
- Implementations- using arrays, using linked lists
- Operation
- Applications
- Queues
- Implementations- using arrays, using a LinkedList, using two stack
- Circular queues
- Priority queues
- Stacks
Week – 04 Trees
A tree is a non-linear data structure where data objects are organised in terms of hierarchical relationships. In this week’s session, we’ll be discussing them one by one.
- In this week, we will discuss about
- Trees.
- Binary trees, their representations
- Pre-order, In-order, post-order traversal
- Binary search trees
- AVL trees
- Trees.
Week – 05 Heaps and Tries
Heap and Trie are special cases of trees. A heap is a special tree-based data structure in which the tree is a complete binary trees.
- In the session, we will discuss about
- How to solve the longest word in the dictionary problem using tries.
- Heaps
- Min Heap
- Max Heap
- Find K largest (or smallest) elements in the array
- Tries
- Longest Word in Dictionary Problem
Week – 06 Graphs & Algorithms
A graph is a data structure that consists of a finite set of vertices, also called nodes and a finite set of ordered pairs of the form (u, v) called edges.
- In this week’s session, We will be discussing different types of algorithms, like:
- Graphs
- Types of graphs
- Breadth-first search, depth-first search
- Topological sort
- Shortest path problem
- Algorithms
- Dijkstra’s algorithm
- Bellman–Ford algorithm
- Minimum spanning tree problem
- Prim’s algorithm
- Kruskal’s algorithm
Week – 07 Sorting
- In this week, we will discuss about
- Sorting-
- Types of sorting techniques
- Bubble sort
- Insertion sort
- Selection sort
- Quick sort
- Merge sort
- Heap sort
- Count sort
- Bucket sort
- Radix sort
- Shell sort
- Types of sorting techniques
- Sorting-
Week – 08 Searching and Hashing
Searching is probably one of the most common actions performed in a regular business application. This involves fetching data stored in data structures like arrays, lists, and maps. Hashing is the process of converting a given key into another value. A hash function is used to generate the new value according to a mathematical algorithm.
- During this week we will be discussing the following:
- Searching
- Linear search
- Binary search
- Linear search
- Hashing
- Hash function
- Collision handling
- Hash function
- Chaining
- Open addressing
- Linear probing, primary clustering
- Quadratic probing, secondary clustering
- Linear probing, primary clustering
- Double hashing
- Searching
Week – 09 Greedy Algorithms
A greedy algorithm is a simple, intuitive algorithm that is used in optimization problems.
- The topics discussed in this session will be-
- The strategy of greedy algorithms
- Elements of greedy algorithms
- Advantages of greedy algorithms
- Disadvantages of greedy algorithms
- Applications of greedy algorithms
- Knapsack problem
- Job sequencing with deadlines
- Huffman coding
Week – 10 Divide and Conquer
The divide and conquer approach is pretty straightforward, the problem at hand is divided into smaller subproblems and then each problem is solved independently. As we keep dividing the subproblems into even smaller problems, we eventually reach a stage where no more division is possible.
- In the session this week, we will discuss:
- The strategy of divide and conquer techniques
- Advantages of divide and conquer techniques
- Disadvantages of divide and conquer techniques
- The master theorem of divide and conquer techniques
- Applications of divide and conquer techniques
- Find the median of two sorted arrays
Week – 11 Backtracking
Backtracking is an algorithmic-technique for solving problems recursively by trying to build a solution incrementally.
- We’ll discuss two methods used to backtrack.
Backtracking-- Brute force approach
- N queens problem
- After backtracking, we will be studying how Java 8 collections framework is implemented. We will be covering the following,
- Set
- List
- Queue
- PriorityQueue
- Map
- HashSet
- TreeSet
- ArrayList
- LinkedList
- Vector
- Dictionary
- Stack
Week – 12 Dynamic Programming
Dynamic Programming is also an algorithmic technique for solving optimization of problems. It is done by breaking the problem down into simpler subproblems, and utilizing the fact that the optimal solution to the overall problem depends upon the optimal solution to its subproblems.
- During this session, we will be covering the following:
- Dynamic programming-
- The strategy of dynamic programming
- Properties of dynamic programming
- Approaches of dynamic programming
- 0/1 knapsack
- Travelling salesman problem
- Floyd Warshall
- In the end, we will conclude and summarize the course by having a brief discussion on
- Greedy vs Divide & Conquer vs Dynamic
- Programming examples
- Dynamic programming-
Course 4 - Introduction to Java Programming 2.0
Week 01 – Basic Constructs of Java Programming Language (Part 1)
- Setting up the environment for Java
- Understanding the Java Ecosystem
- Understanding the Eclipse IDE
- Running your first Java program
- Running and debugging Java Application
Week 02 – Basic Constructs of Java Programming Language (Part 2)
- Data types and operators
- Conditional statements
- Iterative statements
- Other control statements
- Functions and methods
- Working with Arrays in Java
- Working with Strings in Java
Week 03 – Object Oriented Programming (Part 1 )
- Classes and objects
- Constructors
- The ‘this’ pointer
- The static and final keyword
- Access modifiers
Week 04 – Object Oriented Programming (Part 2 )
- Inheritance (is-a relationship)
- Composition (has-a relationship)
- Abstract classes and interfaces
- Method overloading and overriding
- Polymorphism
- Encapsulation and abstraction
Week 05 – Advanced Topics in Java (Part 1)
- Packages
- Exception Handling
- File handling
- I/O
Week 06 – Advanced Topics in Java (Part 2)
- Optionals
- Memory Management
- Multithreading
Course 5 - Everything about Database 2.0
Week 01 – Introduction to Databases
- Introduction to databases
- Downloading XAMPP and creating the first table
- Components of DBMS
- Characteristics of DBMS, ACID properties
- Databases vs File system
- Types of DB
- SQL vs NoSQL
- CRUD operations example in MySQL, OLTP, and OLAP
Week 02 – Creating Database Tables
- Database design
- ER model
- Entity and attributes
- Entity relationships
- Cardinality ratio
- Generalization, specialization, and aggregation
- Case study of Ecommerce website
Week 03 – Introduction to RDBMS
- RDBMS
- Converting ER diagram to table
- Properties of relational model
- Primary key and foreign key
- Relational model
- E-commerce use case
- Codd’s rules
- Data integrity in RDBMS
Week 04 – Database Design
- Database design
- Redundancy
- Insertion anomaly
- Functional dependencies
- Closure set of attributes
- Lossy decomposition
- Dependency preserving
- Normalization
- Transitive dependency
- 3NF
- BCNF
- Multivalued dependency
- 5NF
- Comparison between Normal forms, OLTP, and OLAP
Week 05 – SQL (Part 1)
- Discrete maths
- Relational Algebra and null values
- Relational calculus
- Characteristics of SQL
- Create a DB (example)
- SQL Constraints
- Foreign key
- SQL SELECT statement
- INSERT, DELETE statements
- Scalar and Aggregate functions
- HAVING clause
- SQL Aliases
- IN, BETWEEN operators
- EXISTS, ANY, ALL operator
- Auto increment
- MySQL best practices
Week 06 – SQL (Part 2)
- JOIN-types
- JOIN examples
- String operators
- Compound queries
- SQL index
- SQL views
- Query optimization
- Security Risks
Week 07 – NoSQL
- Introduction to NoSQL
- ACID
- Key value pair-based databases
- Search DB and Graph DB
- Advantages of NoSQL
- Introduction to MongoDB
Week 08 – MongoBD Basics
- Working with MongoDB
- CRUD Operations with examples
- Data Modelling in NoSQL
- One to One and One to Many relationships
- Advanced MongoDB queries
- Join, sort, group, and index
Week 09 – Redis
- In memory DB
- Redis
- Key value store
- Cashing layer
- Datatypes
- Transaction
- Backup of data from Redis
Week 10 – PL/SQL
- PL/SQL Basics
- Operators
- Record
- Recursive Functions
- Exception Handling
- Triggers
Week 11 – More about Databases
- Transactions
- Concurrency control
- Isolation
- Consistency
- ACID
- Database lock
- Database lock types
- Lock Contention
- Deadlock
- Scaling the database
- Storage systems
- Database indexing
- Index types
- Distributed DB
- DB Replication
- Database sharding
- DB on Cloud
Backend Track
On a daily basis we talk to companies expert in these track to fine tune our curriculum. In total, there are 6 courses that are available in this track
Course 1 - Introduction to Java Programming 2.0
Week 01 – Basic Constructs of Java Programming Language (Part 1)
- Setting up the environment for Java
- Understanding the Java Ecosystem
- Understanding the Eclipse IDE
- Running your first Java program
- Running and debugging Java Application
Week 02 – Basic Constructs of Java Programming Language (Part 2)
- Data types and operators
- Conditional statements
- Iterative statements
- Other control statements
- Functions and methods
- Working with Arrays in Java
- Working with Strings in Java
Week 03 – Object Oriented Programming (Part 1 )
- Classes and objects
- Constructors
- The ‘this’ pointer
- The static and final keyword
- Access modifiers
Week 04 – Object Oriented Programming (Part 2 )
- Inheritance (is-a relationship)
- Composition (has-a relationship)
- Abstract classes and interfaces
- Method overloading and overriding
- Polymorphism
- Encapsulation and abstraction
Week 05 – Advanced Topics in Java (Part 1)
- Packages
- Exception Handling
- File handling
- I/O
Week 06 – Advanced Topics in Java (Part 2)
- Optionals
- Memory Management
- Multithreading
Course 2 - Everything about Database 2.0
Week 01 – Introduction to Databases
- Introduction to databases
- Downloading XAMPP and creating the first table
- Components of DBMS
- Characteristics of DBMS, ACID properties
- Databases vs File system
- Types of DB
- SQL vs NoSQL
- CRUD operations example in MySQL, OLTP, and OLAP
Week 02 – Creating Database Tables
- Database design
- ER model
- Entity and attributes
- Entity relationships
- Cardinality ratio
- Generalization, specialization, and aggregation
- Case study of Ecommerce website
Week 03 – Introduction to RDBMS
- RDBMS
- Converting ER diagram to table
- Properties of relational model
- Primary key and foreign key
- Relational model
- E-commerce use case
- Codd’s rules
- Data integrity in RDBMS
Week 04 – Database Design
- Database design
- Redundancy
- Insertion anomaly
- Functional dependencies
- Closure set of attributes
- Lossy decomposition
- Dependency preserving
- Normalization
- Transitive dependency
- 3NF
- BCNF
- Multivalued dependency
- 5NF
- Comparison between Normal forms, OLTP, and OLAP
Week 05 – SQL (Part 1)
- Discrete maths
- Relational Algebra and null values
- Relational calculus
- Characteristics of SQL
- Create a DB (example)
- SQL Constraints
- Foreign key
- SQL SELECT statement
- INSERT, DELETE statements
- Scalar and Aggregate functions
- HAVING clause
- SQL Aliases
- IN, BETWEEN operators
- EXISTS, ANY, ALL operator
- Auto increment
- MySQL best practices
Week 06 – SQL (Part 2)
- JOIN-types
- JOIN examples
- String operators
- Compound queries
- SQL index
- SQL views
- Query optimization
- Security Risks
Week 07 – NoSQL
- Introduction to NoSQL
- ACID
- Key value pair-based databases
- Search DB and Graph DB
- Advantages of NoSQL
- Introduction to MongoDB
Week 08 – MongoBD Basics
- Working with MongoDB
- CRUD Operations with examples
- Data Modelling in NoSQL
- One to One and One to Many relationships
- Advanced MongoDB queries
- Join, sort, group, and index
Week 09 – Redis
- In memory DB
- Redis
- Key value store
- Cashing layer
- Datatypes
- Transaction
- Backup of data from Redis
Week 10 – PL/SQL
- PL/SQL Basics
- Operators
- Record
- Recursive Functions
- Exception Handling
- Triggers
Week 11 – More about Databases
- Transactions
- Concurrency control
- Isolation
- Consistency
- ACID
- Database lock
- Database lock types
- Lock Contention
- Deadlock
- Scaling the database
- Storage systems
- Database indexing
- Index types
- Distributed DB
- DB Replication
- Database sharding
- DB on Cloud
Course 3 - Microservices using Java, Spring & Docker
Week 01 – Introduction to Microservices Architecture
- Types of application architectures
- Comparisons between various architectures
- Introduction to Microservices
- Java Ecosystem for Microservices
- Create a simple microservices
Week 02 – Microservices and Cloud
- Understanding cloud-native app
- Traditional vs cloud-native app
- Understand 12 Factors for cloud-native app
- Sizing and boundary identification for microservices
Week 03 – Creating a Bank Application using Microservices
- Overview of the app we are creating
- Create accounts microservices
- Create cards microservices
- Create loans microservices
Week 04 – Using Docker for Microservices
- Introduction to containerization
- Overview of docker
- Docker image
- Deploy using images/containers
- Build packs
- Docker-compose
Week 05 – Managing Configurations using Spring Cloud
- Configuration management basics
- Sprint cloud configuration
- RefreshScope
- Securing config with encryption
Week 06 – Discovering and Registering to a Service
- Service discovery and registration basics
- Spring cloud config
- Eureka server
Week 07 – Building Resiliency in Microservices
- What is resiliency?
- Bulkhead pattern
- Circuit breaker patterns
- Retry pattern
- Rate Limiter
Week 08 – Cross-Cutting Concerns and Routing in Microservices
- Overview of routing and cross-cutting concerns
- Spring cloud gateway
- Implementing routing
- Implementing cross-cutting concerns – Logging
Week 09 – Challenges with Distributed Logging Aggregation with Monitoring the Health
- Distributed logging/tracing aggregation overview
- Spring cloud sleuth for tracing
- Zipkin overview for logging
- Challenges with monitoring microservices
- Approaches to monitoring microservices
- Using Prometheus and Grafana to monitor
Week 10 – Using Kubernetes for Scaling, Deployment and Self-Recovery
- Kubernetes overview
- K8s cluster
- Deployment
- Self-healing
- Logging/Monitoring of Kubernetes cluster
- Auto-scaling with HPA
- Validating deployed clusters
- Challenges with manually created Kubernetes manifest
Week 11 – Using Helm for Easier Installation and Packaging of YAML Files
- Understand Helm
- Using Helm with Kubernetes cluster
- Creating Helm charts for our microservices
- Some common Helm commands
Week 12 – Spring MVC using Spring Boot
- Overview of Spring MVC
- Spring MVC Architecture
- Using Spring Boot for Spring MVC Configuration
- Performing CRUD operation through a Spring Boot MVC application
Course 4 - Data Structures and Algorithms using JAVA
Week – 01 Introduction
A data structure is a named location that can be used to store and organise data. An algorithm is a collection of steps to solve a particular problem. Data structures provide a means to manage large amounts of data efficiently.
- In the first week of this course, you will learn:
- Stack memory vs heap memory in Java
- Physical vs logical data structure
- Abstract data types and data structures
- Complexity analysis
- Asymptotic analysis, comparison of functions, recurrence relations
- Time complexity
- Space complexity
- Master theorem
- Amortized analysis
- Iteration Vs recursion
- Recursion – Factorial, Fibonacci, finding nCr, tower of Hanoi problem.
Week – 02 Arrays, Strings, and Lists
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. A string is a sequence of characters like – “hello“.
- In this session, we will learn about:
- Arrays
- Static arrays
- Dynamic arrays
- 2D arrays, row-major and column-major mapping, matrices
- Strings
- LinkedList
- Singly LinkedList and its operations
- Doubly LinkedList and its operations
- Circular LinkedList and its operation
- Arrays
Week – 03 Stacks and Queues
The stack is a linear data structure that is used to store a collection of objects. It is based on Last-In-First-Out (LIFO).
- In this session, we will be discussing about:
- Stacks
- Implementations- using arrays, using linked lists
- Operation
- Applications
- Queues
- Implementations- using arrays, using a LinkedList, using two stack
- Circular queues
- Priority queues
- Stacks
Week – 04 Trees
A tree is a non-linear data structure where data objects are organised in terms of hierarchical relationships. In this week’s session, we’ll be discussing them one by one.
- In this week, we will discuss about
- Trees.
- Binary trees, their representations
- Pre-order, In-order, post-order traversal
- Binary search trees
- AVL trees
- Trees.
Week – 05 Heaps and Tries
Heap and Trie are special cases of trees. A heap is a special tree-based data structure in which the tree is a complete binary trees.
- In the session, we will discuss about
- How to solve the longest word in the dictionary problem using tries.
- Heaps
- Min Heap
- Max Heap
- Find K largest (or smallest) elements in the array
- Tries
- Longest Word in Dictionary Problem
Week – 06 Graphs & Algorithms
A graph is a data structure that consists of a finite set of vertices, also called nodes and a finite set of ordered pairs of the form (u, v) called edges.
- In this week’s session, We will be discussing different types of algorithms, like:
- Graphs
- Types of graphs
- Breadth-first search, depth-first search
- Topological sort
- Shortest path problem
- Algorithms
- Dijkstra’s algorithm
- Bellman–Ford algorithm
- Minimum spanning tree problem
- Prim’s algorithm
- Kruskal’s algorithm
Week – 07 Sorting
- In this week, we will discuss about
- Sorting-
- Types of sorting techniques
- Bubble sort
- Insertion sort
- Selection sort
- Quick sort
- Merge sort
- Heap sort
- Count sort
- Bucket sort
- Radix sort
- Shell sort
- Types of sorting techniques
- Sorting-
Week – 08 Searching and Hashing
Searching is probably one of the most common actions performed in a regular business application. This involves fetching data stored in data structures like arrays, lists, and maps. Hashing is the process of converting a given key into another value. A hash function is used to generate the new value according to a mathematical algorithm.
- During this week we will be discussing the following:
- Searching
- Linear search
- Binary search
- Linear search
- Hashing
- Hash function
- Collision handling
- Hash function
- Chaining
- Open addressing
- Linear probing, primary clustering
- Quadratic probing, secondary clustering
- Linear probing, primary clustering
- Double hashing
- Searching
Week – 09 Greedy Algorithms
A greedy algorithm is a simple, intuitive algorithm that is used in optimization problems.
- The topics discussed in this session will be-
- The strategy of greedy algorithms
- Elements of greedy algorithms
- Advantages of greedy algorithms
- Disadvantages of greedy algorithms
- Applications of greedy algorithms
- Knapsack problem
- Job sequencing with deadlines
- Huffman coding
Week – 10 Divide and Conquer
The divide and conquer approach is pretty straightforward, the problem at hand is divided into smaller subproblems and then each problem is solved independently. As we keep dividing the subproblems into even smaller problems, we eventually reach a stage where no more division is possible.
- In the session this week, we will discuss:
- The strategy of divide and conquer techniques
- Advantages of divide and conquer techniques
- Disadvantages of divide and conquer techniques
- The master theorem of divide and conquer techniques
- Applications of divide and conquer techniques
- Find the median of two sorted arrays
Week – 11 Backtracking
Backtracking is an algorithmic-technique for solving problems recursively by trying to build a solution incrementally.
- We’ll discuss two methods used to backtrack.
Backtracking-- Brute force approach
- N queens problem
- After backtracking, we will be studying how Java 8 collections framework is implemented. We will be covering the following,
- Set
- List
- Queue
- PriorityQueue
- Map
- HashSet
- TreeSet
- ArrayList
- LinkedList
- Vector
- Dictionary
- Stack
Week – 12 Dynamic Programming
Dynamic Programming is also an algorithmic technique for solving optimization of problems. It is done by breaking the problem down into simpler subproblems, and utilizing the fact that the optimal solution to the overall problem depends upon the optimal solution to its subproblems.
- During this session, we will be covering the following:
- Dynamic programming-
- The strategy of dynamic programming
- Properties of dynamic programming
- Approaches of dynamic programming
- 0/1 knapsack
- Travelling salesman problem
- Floyd Warshall
- In the end, we will conclude and summarize the course by having a brief discussion on
- Greedy vs Divide & Conquer vs Dynamic
- Programming examples
- Dynamic programming-
Course 5 - The Complete Front-End Development - 2.0
Module 01 – HTML5
- The topics covered in this module are:
- Valid document structure, anatomy of HTML syntax
- HTML5 semantic tags – Elements and attributes, Block and inline elements
- Essential HTML5 tags – headings, paragraph, styles, comments, colours
- HTML lists – unordered and ordered lists
- Inserting images using HTML
- How to create hyperlinks using anchor tags
- HTML tables
- HTML forms
- HTML Div – Layout and separate content for CSS styling
- Classes and ID
- HTML iFrames
- HTML Graphics
- HTML Media
- HTML APIs- Geolocation, Web Storage, Web Workers, Drag and Drop, SS Event
- HTML best practices- HTML vs XHTML”
Module 02 – CSS
- In this module, you will learn:
- Basics of CSS3, CSS rules
- Comments, Colors, background, border, margin, padding, height, and width
- CSS selectors and properties
- Inline, internal, and external CSS
- Font styling, web safe fonts – Texts, icons, links
- The ‘Box model’
- CSS sizing methods
- CSS static, relative, and absolute positioning systems
- CSS float and clear
- Pseudo classes and pseudo elements
- Class vs. ID
- Opacity, Navigation bars, Dropdowns
- CSS rule conflict resolution. specificity, and implementing style hierarchy
- Images and forms styling
- Transitions, animations, pagination, 2D and 3D transforms
- Responsive web design
- The grid system
- Flexbox, media queries
- CSS coding best practices
Module 03 – Bootstrap
- In this module you will learn:
- The mobile-first paradigm
- Wireframes in the design phase
- Twitter Bootstrap – Grid System
- How to install the Bootstrap framework
- Bootstrap containers to layout website easily
- Other Bootstrap components
- Bootstrap carousels
- Bootstrap cards
- Bootstrap navigation bars
- Bootstrap themes
- Font-awesome
Module 04 – Page Events
- The topics covered in this module are:
- ECMAScript
- JavaScript vs Java
- Popularity and Importance
- Basics of JavaScript
- JS Statements
- JS functions
- Adding JS to your website
- Document Object Model
- Manipulating DOM
- Events
- Exception Handling
- Forms and JS
Module 05 – JavaScript
- The topics covered in this module are:
- Variables, data types, and operators in JavaScript
- Statements, syntax, comments, and events
- Loops, control, and conditionals
- JS forms
- Document Object Model (DOM)
- Browser Object Model (BOM)
- JS functions
- Scope and closures
- Object-Oriented Programming (OOP)
- JS objects and prototypes – keyword
- Cookies
- Exception handling
- Arrow functions
- Promises
- Template string, default parameters, spread and rest operator, destructuring, generators, Set, Map, WeakSet, WeakMap
- HTML APIs
- Separation of concerns, refactoring, debugging, and coding best practices
Module 06 – AJAX, JSON and XML
- We will be covering
- Serving and retrieving data to a website
- Asynchronous loading – Set up and handle AJAX requests and responses
- JSON
- XML
- Processing JSON data
- JSON vs. XML
Our courses have been designed by industry experts to help students achieve their dream careers
Companies where our students got jobs
Whether you’re looking to start a new career, or change your current one, Skill-Lync helps you get ready to get placed in Top Companies.
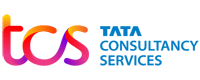
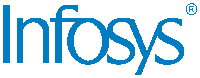


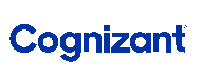
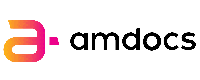

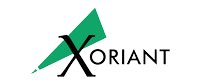




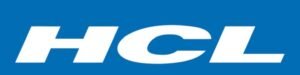

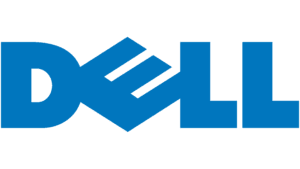
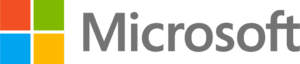
A tailored and adaptive certification program designed to empower tech professionals in propelling their careers forward.
This comprehensive Post Graduate Program in Full Stack Web Development delves into a spectrum of crucial topics including JavaScript, SQL, algorithms, databases, and web applications. Upon completion, all participants receive certification, with the top 5% distinguished by a merit certificate.
Tech-Lync’s Post Graduate Program in Full Stack Web Development is one of the best full-stack developer courses online. This 24-week long program equips you with information on how to handle a website. If you are interested in full-stack development jobs, freelancing, and product ownership opportunities, then this course is for you.
Who Should Take this Post Graduate Program in Full Stack Web Development?
This Post Graduate Program is suitable for students with a degree in B.E/ M.E/B.Tech/M.Tech in IT, CSE, EEE, ECE, Mechanical, or Civil department. This program can be taken by engineers and experienced working professionals willing to switch to the full-stack development field. This program offers career support services to help you get placed after the completion of the course. It empowers you to use back-end and front-end technologies in building interactive and responsive web applications.
If you are interested in making your career as a full-stack developer, back-end or front-end developer, or web designer, this program is apt for you. There is a huge demand in the industry for the skills you will be trained on during this course.
What Will You Learn?
This Post Graduate program will teach you how you can become a front-end developer. Further, it will take you through the process of building an exceptional user interface through React or Angular.
This program will help you grasp the fundamental layers and understand how they will scale as the website traffic grows. The main topics of discussion revolve around these imperative layers like the front-end, database, and back-end. Out of all the courses for a full stack developer, this Post Graduate Program in Full Stack Web Development brings several career opportunities and massive growth prospects for you after its completion. With nine industry-level projects, the course will equip you with full-stack developer skills essential to kick-start your career.
One of the best full-stack web development courses, it provides you with multiple opportunities to work on industry-relevant projects in Data Structures and Algorithms using JAVA, Front-End Development, Java Programming, Microservices using Java, Spring & Docker, and Angular Web Development. This full-stack course helps learners to master advanced tools in web development through real-time project experience. Having a well-defined project portfolio is also integral to boosting your chances of being noticed by recruiters as a versatile candidate. Aspiring engineers must undergo training through project-oriented full-stack web developer courses like this to establish themselves as job-ready professionals.
Skills You Will Gain
- Web application development skills
- Knowledge of CSS
- Knowledge of MongoDB
- SQL knowledge
- Knowledge of algorithms
- Programming skills
- Database knowledge
Key Highlights of the Program
- You will be recognized with a certificate of completion after the course. If you are a meritorious student and come in the top 5% of learners, you will be eligible for a merit certificate.
- You will get Individual Video Support, Group Video Support, Email Support, and Forum Support to clear your queries and doubts.
- Real-time industry-relevant projects will make your learning purposeful.
- Also, our Placement Support Team will help you to carve your resume and will train you with mock interviews.
Why should you consider undertaking our Full-Stack Web Development training?
Tech-Lync’s web development course focuses on imparting the key technical skills required by the IT industry. We constantly revise our full-stack course curriculum to make it aligned with the new trends that are being discovered.
We provide one of the full-stack online training in India for engineers like you, with a mission to level up the engineering workforce. This full-stack training online delves deep into the essential technicalities and teaches the most in-demand software tools that industry professionals use.
Combined with our unique pedagogy, this online course for full-stack developers can help you either kick-start or advance your career if you are an aspiring professional.
Career Opportunities after Taking the Course
You can opt for a higher degree in the same field of study or choose a career as:
- Full-Stack Developer – There is a huge demand in the industry for the skills you will be trained on during this course.
- Back-End Developer – You will be able to apply your server-side language skills like Java, Ruby, Python, and PHP and professionally work on the brain of any technology used by an organization.
- Front-End Developer – With your learning enhanced in JavaScript, HTML, and CSS, you can choose to become a front-end developer engaged in web designing.
- Web Developer – A mix of both – front end and back end with proficiency in languages like Ruby, Python, etc., can help you get into web development.
- Web Designer – You will be working extensively in developing and maintaining the website.
FAQs on this Post Graduate Program in Full Stack Web Development
1, Is there a placement for learners at the end of the course?
There are abundant opportunities offered after completing the course, but there is no guarantee of placement. Services and several chances are made available to you looking for a way to get into tech biggies.
2, While completing the Post Graduate Program in Full Stack Web Development by Tech-Lync, what are the different career support services that the learners will get?
You will get several career support services like:
- Enhancement of technical skills
- Mock interviews
- Resume building
- LinkedIn support
3, How many modules are there in the Post Graduate Program in Full Stack Web Development?
There are six modules to complete in the full-stack web development course.
4, What is the approximate salary range that can be expected after completing the Post Graduate Program in Full Stack Web Development?
After completing the Java full stack developer course, you can expect a salary ranging from Rs. 2.5 – 6 LPA.
6, What kind of companies can the learners get a job in?
You can get a job in various services ranging from consultancy to Y-Combinator start-ups.
7, Is there placement support for this full-stack developer course?
Yes, as one of the best full-stack developer courses it offers career guidance and placement support.
Program Fees
Connect with our career counselors to explore flexible payment options that suit your financial needs.
INR 60,000
Inclusive of all charges
Achieve Job Readiness with Our Extensive Industry-Aligned Program for Fresh Graduates & Early Career Professionals
Low cost EMIs and full payment discount available
EMIs starting
INR 10,000/month
- Career Services Assurance with access to our extensive network of 500+ recruitment partners for abundant interview opportunities
- Personalized Career Services Mentorship, including Resume Building, LinkedIn Profile Optimization, Mock Interviews, Communication & Aptitude Training, and an Interview Practice Toolkit.
- 5 Years Accessto Tech-Lync’s Learning Management System (LMS)
- Personalized Page to showcase Projects & Certifications
Showcase Your Projects & Certifications on a Personalized Page
Live Individual & Group Sessions to Address Queries, Discuss Progress, and Plan Studies.
- 3-Month Unpaid Internship at Tech-Lync Focused on Industry-Oriented Projects.
- Personalized and hands-on support via email and telephone ensures prompt query resolution and continuous monitoring of learner progress.
- Gain access to a job-oriented, industry-relevant curriculum curated by global industry experts, complemented by interactive live sessions.
Full Fee Upfront Payment
EMI Payment Plans
PAYMENT TYPE | DURATION | INSTALLMENT |
---|---|---|
Zero Cost EMI | 3 Months | ₹20,000 |
6 Months | ₹10,000 | |
EMI Loan Plans | 12 Months | ₹5,000* |
22 Months | ₹2,727* |
*Inclusive of Interest
Instructors profiles
Our courses are designed by leading academicians and experienced industry professionals.
9 industry experts
Our instructors are industry experts along with a passion to teach.
6 - 12 years in the experience range
Instructors with 6 – 12 years extensive industry experience.
Areas of expertise
- Full Stack development
- Cyber Security
- Computer Science
- Lean Six Sigma Black Belt
- Angular Framework
- JavaScript
- React Framework
Got more questions?
Talk to our Team Directly
Please fill in your number & an expert from our team will call you shortly.
Tech-Lync is dedicated to providing advanced engineering courses that are directly relevant to industry needs, bridging the gap between academic knowledge and practical skills.